Programmer's Python
Everything is an Object
Buy Now: from your local Amazon
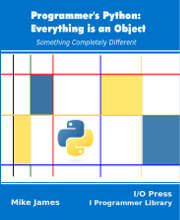
Errata: None
This book sets out to explain the deeper logic in the approach that Python 3 takes to classes and objects. The subject is roughly speaking everything to do with the way Python implements objects. That is, in order of sophistication, metaclass; class; object; attribute; and all of the other facilities such as functions, methods and the many “magic methods” that Python uses to make it all work. This is a fairly advanced book in the sense that you are expected to know basic Python. However, it tries to explain the ideas using the simplest examples possible. As long as you can write a Python program, and you have an idea what object-oriented programming is about, it should all be understandable and, as important, usable. This is not a cookbook and there are no complete examples of real programs – that’s your assignment. This is also a focused book in the sense that there are large parts of Python it doesn’t cover. For example, it doesn’t deal with program structure, concurrency and data structures - these will be covered in companion volumes.
Contents
Chapter 1
Getting Started 13
Getting a Python 15
Strings 17
Data Structures 18
Programs in IDLE 19
Control Structures – Loops 20
Space Matters 22
Conditionals and Indenting 23
Everything Is An Object – References 25
Functions 26
An Object of My Own 28
Inheriting the Point 30
Main & Modules 31
IDEs for Python 32
Pythonic – The Meta Philosophy 33
Where Next 34
Summary 35
Chapter 2
Variables, Objects and Attributes 37
Variables and Objects 37
Dynamic Attributes 40
Nested Objects 42
Namespaces and Modules 42
More Modules 44
Namespace Access – Advanced Topic 45
Where Next 46
Summary 47
Chapter 3
The Function Object 49
The Function Definition 49
Pass-by-Object Reference 50
Functions are Objects 51
Why Are Functions Objects? 52
Lambda Expressions 53
Anonymous Functions 54
Function Self Reference 54
Summary 56
Chapter 4
Scope, Lifetime and Closure 57
Global v Local 57
Inner Functions 59
Local, Global and Nonlocal 59
Closure 61
A Common Error 65
What Use is Closure? 66
Closures __closure__ and Cells (Advanced) 68
Summary 71
Chapter 5
Advanced Functions 73
Default Parameters 73
Keyword Parameters 74
Variable Parameters 75
Unpacking Into Parameters 77
Return Values 78
Docstrings and Annotations 80
Decorators 82
Making the Wrap Transparent – functools 84
Multiple Decorators 86
Decorator Factories 86
Summary 89
Chapter 6
Class, Methods and Constructors 91
The Classical Constructor 91
The Class Object 92
Instance Attributes 93
What is a Method? 94
How Functions Become Methods 95
Bind Early, Bind Often 98
Class Binds 100
Other Bindings 101
The Initializer __init__ 103
Multiple Constructors 104
Class for Methods, Instance for Data 105
Summary 106
Chapter 7
Inside Class 107
__new__ and __init__ 107
Singleton 110
Customizing Immutable Data Objects 111
How Instances Become Functions 112
Summary 113
Chapter 8
Meeting Metaclass 115
Metaclass Basics 115
Class Creation Using Type 116
Meta __new__ and __init__ 118
__prepare__ 120
An Example – Final Classes 121
Meta Attributes and Methods 122
How Classes become Functions – Metaclass __run__ 123
Singleton Revisited 124
Abstract Base Class 125
Decorators Revisited 127
Singleton as Decorator 132
Using Metaclass 133
Summary 134
Chapter 9
Advanced Attributes 135
Private Attributes and Name Mangling 135
Private Attributes and Object Factories 136
Properties 138
Private Properties 141
Property as Decorator 143
Summary 145
Chapter 10
Custom Attribute Access 147
The Descriptor Object 147
Custom Attribute Access 150
Customizing Assignment __setattr__ 153
Default Methods 155
Custom Deleting Attributes 155
Custom Dir 156
Slots 157
Index/Key Access 160
Summary 162
Chapter 11
Single Inheritance 163
Code Reuse 163
Object-Oriented Philosophies 165
Basic Inheritance 166
Overriding 167
Simple Super() 167
Super super() and the mro 169
Inheriting Instance Attributes 172
Do We Need to Use super? 174
Customizing Subclassing 174
Metaclass Inheritance 176
Summary 179
Chapter 12
Multiple Inheritance 181
Why Multiple Inheritance? 181
The Problem with Multiple Inheritance 184
Linearization 185
The C3 Algorithm and the mro 186
Computing the C3 mro 188
Getting to Know Linearization 190
Calling Super Cooperative Multiple Inheritance 192
The Dwindling Parameter Pattern 195
Summary 199
Chapter 13
Class and Type 201
Three Types Of Type 201
Primitive Data Types 202
Class-Based Type 204
Hierarchical Typing 205
Inheritance as the Model 206
Python and Type 207
Instance and Subclass 208
Duck Typing 210
Summary 212
Chapter 14
Type Annotation 213
Strong Typing 213
Gradual Typing in Python- Type Hints 215
Subtype 216
Simple Types 216
Complex Types 219
The Callable – a Complex Type 220
Custom Generics 221
Restricted Type Variables and Generic Functions 223
Co and Contravariance 225
Co & Contravariant Generics 228
Reflections on Type Annotations 231
How Type Annotations Work 231
Summary 233
Chapter 15
Operator Overloading 235
Arithmetic Expressions 235
The Arithmetic Operators 236
Type Conversion, Rounding 237
Custom Comparisons 238
Hashing 239
Object Representation 242
Summary 244
About the Author
Mike James is editor of I-Programmer.info, an online magazine written by programmers for programmers. He has a BSc in Physics, an MSc in Mathematics and a PhD in Computer Science. As an author he has published dozens of books, the latest being The Programmers Guide to Kotlin, Android Programming in Kotlin and Android Programming in Java.
Buy Now: from your local Amazon