Programming The ESP32 In C
Using The Arduino Library
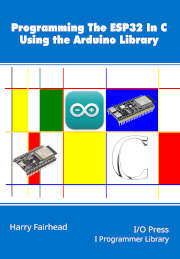
We have decided not to make the programs available as a download because this is not the point of the book - the programs are not finished production code but something you should type in and study.
The best solution is to provide the source code of the programs in a form that can be copied and pasted into a VS Code project.
The only downside is that you have to create a project to paste the code into.
To do this follow the instruction in the book.
All of the programs below were copy and pasted from working programs in the IDE. They have been formatted using the built in formatter and hence are not identical in layout to the programs in the book. This is to make copy and pasting them easier. The programs in the book are formatted to be easy to read on the page. Some of the programs are presented complete even though they were presented in the book as fragments.
Important Note For NANO ESP32 users:
All of the programs make use of GPIO numbering not Arduino numbering.
To change the way that the IDE interprets GPIO numbers use the menu command
Tools,Pin Numbering and select By GPIO number (legacy)
After this all of the programs will work on the NANO ESP32 with minor modification to the GPIO lines used.
Notice that the NANO ESP32 currently doesn't implment some ESP32 features.
These ommissions and changes are noted along with the code.
If anything you consider important is missing or if you have any requests or comments contact:
This email address is being protected from spambots. You need JavaScript enabled to view it.
Page 42
void setup() {
pinMode(2, OUTPUT);
}
void loop() {
digitalWrite(2, HIGH);
delay(1000);
digitalWrite(2, LOW);
delay(1000);
}
Page 44
void setup() {
pinMode(2, OUTPUT);
}
void gpio_toggle(int gpio_num) {
digitalWrite(gpio_num, !digitalRead(gpio_num));
}
void loop() {
gpio_toggle(2);
delay(1000);
gpio_toggle(2);
delay(1000);
}
Page 48
void setup() {
pinMode(2, OUTPUT);
}
void loop() {
digitalWrite(2, HIGH);
digitalWrite(2, LOW);
}
void setup() {
pinMode(2, OUTPUT);
}
volatile int waste;
void loop() {
digitalWrite(2, HIGH);
for(waste=0;waste<6;waste++);
digitalWrite(2, LOW);
}
Page 50
void setup() {
pinMode(2, OUTPUT);
}
void loop() {
digitalWrite(2, HIGH);
delayMicroseconds(1);
digitalWrite(2, LOW);
delayMicroseconds(1);
}
Page 50
void setup() {
pinMode(2, OUTPUT);
}
volatile int i = 0;
int n;
void loop() {
for (n = 1; n < 11; n++) {
digitalWrite(2, HIGH);
for (i = 0; i < n; i++) {}
digitalWrite(2, LOW);
for (i = 0; i < n; i++) {}
}
}
Page 52
unsigned long tick_us(unsigned long offset) {
return micros() + offset;
}
void delay_until(unsigned long t) {
do {
} while (t > micros());
}
void setup() {
pinMode(2, OUTPUT);
}
volatile int i = 0;
int n = 2;
unsigned long width = 1000;
unsigned long t;
void loop() {
t = tick_us(width);
digitalWrite(2, HIGH);
for (i = 0; i < n; i++) {}
delay_until(t);
t = tick_us(width);
digitalWrite(2, LOW);
for (i = 0; i < n; i++) {}
delay_until(t);
}
Page 54
void setup() {
pinMode(2, OUTPUT);
pinMode(4, OUTPUT);
}
void loop() {
digitalWrite(2, HIGH);
digitalWrite(4, LOW);
digitalWrite(2, LOW);
digitalWrite(4, HIGH);
}
Page 56
void gpio_set(int32_t value, int32_t mask) {
int32_t *OutAdd = (int32_t *)GPIO_OUT_REG;
*OutAdd = (*OutAdd & ~mask) | (value & mask);
}
void setup() {
pinMode(2, OUTPUT);
pinMode(4, OUTPUT);
}
volatile int i = 0;
int32_t mask = 1 << 2 | 1 << 4;
int32_t value1 = 0 << 2 | 1 << 4;
int32_t value2 = 1 << 2 | 0 << 4;
void loop() {
gpio_set(value1, mask);
for (i = 0; i < 5; i++) {}
gpio_set(value2, mask);
}
Page 80
void setup() {
pinMode(2, OUTPUT);
pinMode(4, INPUT_PULLUP);
}
void loop() {
if (digitalRead(4)) {
digitalWrite(2, 1);
} else {
digitalWrite(2, 0);
delay(500);
}
}
Page 82
void setup() {
pinMode(2, OUTPUT);
pinMode(4, INPUT_PULLUP);
}
void loop() {
while (digitalRead(4) == 1) {};
delay(10);
while (digitalRead(4) == 0) {};
digitalWrite(2, 1);
delay(1000);
digitalWrite(2, 0);
}
Page 83
unsigned long tick_ms(unsigned long offset) {
return millis() + offset;
}
void setup() {
pinMode(2, OUTPUT);
pinMode(4, INPUT_PULLUP);
}
void loop() {
while (digitalRead(4) == 1) {};
int t = tick_ms(2000);
delay(10);
while (digitalRead(4) == 0) {};
if (tick_ms(0) < t) {
digitalWrite(2, 1);
delay(1000);
digitalWrite(2, 0);
} else {
for (int i = 0; i < 10; i++) {
digitalWrite(2, 1);
delay(1000);
digitalWrite(2, 0);
delay(1000);
}
}
}
Page 84
unsigned long tick_us(unsigned long offset) {
return micros() + offset;
}
void setup() {
pinMode(4, INPUT);
Serial.begin(9600);
}
void loop() {
while (digitalRead(4) == 1) {};
while (digitalRead(4) == 0) {};
int t = tick_us(0);
while (digitalRead(4) == 1) {};
t = tick_us(0) - t;
Serial.println(t);
delay(1000);
}
Page 87
unsigned long tick_us(unsigned long offset) {
return micros() + offset;
}
void delay_until(unsigned long t) {
do {
} while (t > micros());
}
void setup() {
pinMode(4, INPUT_PULLUP);
Serial.begin(9600);
}
unsigned long t, tpush, twait;
int s = 0;
int i;
void loop() {
i = digitalRead(4);
t = tick_us(0);
switch (s) {
case 0: //button not pushed
if (!i) {
s = 1;
tpush = t;
}
break;
case 1: //Button pushed
if (i) {
s = 0;
if ((t - tpush) > 2000000) {
Serial.println("Button held");
} else {
Serial.println("Button pushed");
}
}
break;
default:
s = 0;
}
delay_until(t + 100 * 1000);
}
Page 89
unsigned long tick_us(unsigned long offset) {
return micros() + offset;
}
void delay_until(unsigned long t) {
do {
} while (t > micros());
}
void setup() {
pinMode(4, INPUT_PULLUP);
Serial.begin(9600);
}
unsigned long t, tpush, twait;
int s = 0;
int i;
void loop() {
i = digitalRead(4);
t = tick_us(0);
switch (s) {
case 0: //button not pushed
if (!i) {
s = 1;
tpush = t;
}
break;
case 1: //Button pushed
if (i) {
s = 0;
if ((t - tpush) > 2000000) {
Serial.println("Button held");
} else {
Serial.println("Button pushed");
}
}
break;
default:
s = 0;
}
delay_until(t + 100 * 1000);
}
Page 91 Note Change GPIO 16 to GPIO 18 for the NANO ESP32
unsigned long tick_us(unsigned long offset) {
return micros() + offset;
}
void delay_until(unsigned long t) {
do {
} while (t > micros());
}
unsigned long t;
int edge;
int buttonNow, buttonState;
int s = 0;
void setup() {
pinMode(4, INPUT_PULLUP);
buttonState = digitalRead(4);
pinMode(2, OUTPUT);
pinMode(16, OUTPUT);
pinMode(17, OUTPUT);
digitalWrite(2, 1);
digitalWrite(16, 0);
digitalWrite(17, 0);
Serial.begin(9600);
}
void loop() {
buttonNow = digitalRead(4);
t = tick_us(0);
edge = buttonNow-buttonState;
buttonState = buttonNow;
switch (s) {
case 0:
if (edge == 1) {
s = 1;
digitalWrite(2, 0);
digitalWrite(16, 1);
digitalWrite(17, 0);
}
break;
case 1:
if (edge == 1) {
s = 2;
digitalWrite(2, 0);
digitalWrite(16, 0);
digitalWrite(17, 1);
}
break;
case 2:
if (edge == 1) {
s = 0;
digitalWrite(2, 1);
digitalWrite(16, 0);
digitalWrite(17, 0);
}
break;
default:
s = 0;
}
delay_until(t + 100 * 1000);
}
Page 98
void ISR(void){
digitalWrite(2, !digitalRead(2));
}
void setup() {
pinMode(2, OUTPUT);
pinMode(4, INPUT_PULLUP);
attachInterrupt(digitalPinToInterrupt(4), ISR, CHANGE);
}
void loop() {}
Page 99 Note Change GPIO 16 to GPIO 18 for the NANO ESP32
void IRAM_ATTR ISR(void) {
digitalWrite(2, !digitalRead(2));
}
void setup() {
pinMode(2, OUTPUT);
pinMode(4, INPUT_PULLUP);
attachInterrupt(digitalPinToInterrupt(4), ISR, CHANGE);
pinMode(16, INPUT_PULLUP);
attachInterrupt(digitalPinToInterrupt(16), ISR, CHANGE);
}
void loop() {}
Page 101
volatile unsigned long t = 0;
volatile int state = 0;
unsigned long tick_us(unsigned long offset) {
return micros() + offset;
}
void ISR(void) {
t = tick_us(-t);
state++;
digitalWrite(2, !digitalRead(2));
}
void setup() {
pinMode(2, OUTPUT);
pinMode(4, INPUT);
attachInterrupt(digitalPinToInterrupt(4), ISR, CHANGE);
Serial.begin(9600);
}
void loop() {
while (state != 2) {};
detachInterrupt(digitalPinToInterrupt(4));
Serial.print(t);
Serial.print(" ");
Serial.println(state);
state = 0;
t = 0;
delay(1000);
attachInterrupt(digitalPinToInterrupt(4), ISR, CHANGE);
}
Page 102
volatile char data[3];
void ISR(void) {
for (int i = 0; i < 3; i++) data[i] = 'A';
}
void setup() {
pinMode(4, INPUT);
attachInterrupt(digitalPinToInterrupt(4), ISR, CHANGE);
Serial.begin(9600);
}
void loop() {
for (int i = 0; i < 3; i++) data[i] = 'B';
if (data[0] != data[1] || data[1] != data[2]
|| data[2] != data[0]) {
Serial.print(data[0]);
Serial.print(data[1]);
Serial.println(data[2]);
}
}
Page 103
volatile char data[3];
void ISR(void) {
for (int i = 0; i < 3; i++) data[i] = 'A';
}
void setup() {
pinMode(4, INPUT);
attachInterrupt(digitalPinToInterrupt(4), ISR, CHANGE);
Serial.begin(9600);
}
void loop() {
detachInterrupt(digitalPinToInterrupt(4));
for (int i = 0; i < 3; i++) data[i] = 'B';
if (data[0] != data[1] || data[1] != data[2]
|| data[2] != data[0]) {
Serial.print(data[0]);
Serial.print(data[1]);
Serial.println(data[2]);
}
attachInterrupt(digitalPinToInterrupt(4), ISR, CHANGE);
}
Page 104
Note NANO ESP32 currently uses a different timerBegin
that demands that you set the timer number, divider and count up
hw_timer_t * timerBegin(uint8_t num, uint16_t divider, bool countUp)
To make the program work change the line timer = timerBegin(1221); to read
timer = timerBegin(0,65535,true);
hw_timer_t *timer;
uint64_t count;
void setup() {
timer = timerBegin(1221);
Serial.begin(9600);
}
void loop() {
do {
count = timerRead(timer);
} while (count < 1000);
timerStop(timer);
Serial.println(count);
timerStart(timer);
}
Page 105
Note NANO ESP32 currently uses a different timerBegin
that demands that you set the timer number, divider and count up
hw_timer_t * timerBegin(uint8_t num, uint16_t divider, bool countUp)
To make the program work change the line return timerBegin(1000000); to read
return timerBegin(0,80,1);
hw_timer_t *timer;
hw_timer_t *tick_us_start(void) {
return timerBegin(1000000);
}
int64_t tick_us(hw_timer_t *timer, int64_t offset) {
return timerRead(timer) + offset;
}
void setup() {
timer = tick_us_start();
Serial.begin(9600);
}
void loop() {
int64_t tick = tick_us(timer, 1000000);
while (tick_us(timer, 0) < tick) {};
Serial.println(tick_us(timer, 0));
}
Page 106 Currently not supported on the NANO ESP32
hw_timer_t* timer;
void ISR(void* user_data) {
digitalWrite(2, !digitalRead(2));
}
void setup() {
pinMode(2, OUTPUT);
timer = timerBegin(0,8000,true);
timerAlarm(timer, 1000, true, 0);
timerAttachInterruptArg(timer, ISR, NULL);
}
Page 111
void setup() {
}
void loop() {
for (int d = 0; d < 256; d++) {
analogWrite(2, d);
delay(10);
}
}
Page 112
void setup() {
}
void loop() {
for (int d = 0; d < 256; d++) {
analogWrite(2, d * d * d / 255 / 255);
delay(10);
}
}
Page 114
uint8_t wave[256];
void setup() {
for (int i = 0; i < 256; i++) {
wave[i] = (uint8_t)((128.0 + sinf((float)i * 2.0 *
3.14159 / 255.0) * 128.0));
}
}
void loop() {
for (int i = 0; i < 256; i++) {
analogWrite(2, wave[i]);
delayMicroseconds(1000);
}
}
Page 121 The LEDC library is not currently supported by the Arduino NANO ESP32
void setup() {
ledcAttach(2, 1000, 8);
}
void loop() {
for (int d = 0; d < 256; d++) {
ledcWrite(2, d);
delay(10);
}
}
Page 121 The LEDC library is not currently supported by the Arduino NANO ESP32
void setup() {
ledcAttach(2, 1000, 8);
ledcAttach(4, 1000, 8);
}
void loop() {
for (int d = 0; d < 256; d++) {
ledcWrite(2, d);
ledcWrite(4, 255-d);
delay(10);
}
}
Page 123 The LEDC library is not currently supported by the Arduino NANO ESP32
uint8_t wave[256];
void setup() {
ledcAttach(2, 150000, 8);
for (int i = 0; i < 256; i++) {
wave[i] = (uint8_t)((128.0 +
sinf((float)i * 2.0 * 3.14159 / 255.0) * 128.0));
}
}
void loop() {
for (int i = 0; i < 256; i++) {
analogWrite(2, wave[i]);
delayMicroseconds(7);
}
}
Page 124
The LEDC library is not currently supported by the Arduino NANO ESP32
void setup() {
ledcAttach(2, 10000, 8);
ledcWriteTone(2, 262);
}
void loop() {
}
Page 125
The LEDC library is not currently supported by the Arduino NANO ESP32
void setup() {
ledcAttach(2, 1000, 8);
ledcFade(2, 0, 255, 1000);
}
void loop() {
}
Page 126
The LEDC library is not currently supported by the Arduino NANO ESP32
volatile int fading;
void ISR(void) {
fading = false;
}
void setup() {
ledcAttach(2, 1000, 8);
ledcFadeWithInterrupt(2, 0, 255, 1000, ISR);
fading = true;
}
void loop() {
if (!fading) {
delay(100);
ledcFadeWithInterrupt(2, 0, 255, 1000, ISR);
fading = true;
}
}
Page 127
The LEDC library is not currently supported by the Arduino NANO ESP32
#include "driver/ledc.h"
void setup() {
ledcAttach(2, 1000, 8);
ledcAttach(4, 1000, 8);
ledcWrite(2, 128);
ledcWrite(4, 128);
ledc_set_duty_with_hpoint(LEDC_LOW_SPEED_MODE,
LEDC_CHANNEL_1, 128,64);
}
void loop() {
}
Page 137
class UniMotor {
private:
int gpio;
int duty;
public:
UniMotor(int pin) {
gpio = pin;
}
void setSpeed(float s) {
duty = s * 255;
analogWrite(gpio, duty);
}
};
UniMotor *motor1;
void setup() {
motor1 = new UniMotor(2);
motor1->setSpeed(0.5);
}
void loop() {
}
Page 142
class BiMotor {
private:
int gpio1;
int gpio2;
int duty;
bool forward;
public:
BiMotor(int pin1, int pin2) {
gpio1 = pin1;
gpio2 = pin2;
}
void setSpeedDirection(float s, bool f) {
duty = s * 255;
forward = f;
if (forward) {
analogWrite(gpio1, 0);
analogWrite(gpio1, duty);
} else {
analogWrite(gpio2, 0);
analogWrite(gpio2, duty);
}
}
};
BiMotor *motor1;
void setup() {
motor1 = new BiMotor(2, 4);
motor1->setSpeedDirection(0.5, false);
}
void loop() {}
Page 145
The LEDC library is not currently supported by the Arduino NANO ESP32
class Servo {
private:
int gpio;
int max;
int min;
public:
Servo(int pin) {
gpio = pin;
max = (2 << 9) * 12.5 / 100;
min = (2 << 9) * 2.5 / 100;
ledcAttach(pin, 50, 10);
}
void setAngle(float a) {
int duty = (float)(max - min) * a + min;
ledcWrite(2, duty);
}
};
void loop() {
servo1->setAngle(1.0);
delay(100);
servo1->setAngle(0.5);
delay(100);
servo1->setAngle(0.0);
delay(100);
}
Page 153
Use GPIO 5, 6, 7 and 8 for the Arduino NANO ESP32
#include <Stepper.h>
Stepper stepMot1 = Stepper(200, 16, 17, 18, 19);
void setup() {
stepMot1.setSpeed(1);
stepMot1.step(100);
}
void loop() {
}
Page 157
Use GPIO 5, 6, 7 and 8 for the Arduino NANO ESP32
change Stepperbi stepper1=Stepperbi(16); to Stepperbi stepper1=Stepperbi(5);
class Stepperbi {
private:
int gpio;
int stepTable[8] = { 1, 0, 2, 1, 3, 2, 0, 3 };
int state = 0;
public:
Stepperbi(int pin1) {
gpio = pin1;
for (int i = 0; i < 4; i++) {
pinMode(gpio + i, OUTPUT);
digitalWrite(gpio + i, 0);
}
digitalWrite(gpio, 1);
}
void step(int step) {
if (step > 0) {
digitalWrite(gpio + stepTable[state], (state + 1) % 2);
state = (state + 1) % 8;
} else {
state = (state +7) % 8;
digitalWrite(gpio + stepTable[state], state % 2);
};
}
};
Stepperbi stepper1=Stepperbi(16);
void setup(){};
void loop() {
stepper1.step(1);
delay(10);
}
Page 157
Use GPIO 5, 6, 7 and 8 for the Arduino NANO ESP32
change Stepperbi stepper1=Stepperbi(16); to Stepperbi stepper1=Stepperbi(5);
class Stepperbi {
private:
int gpio;
int stepTable[4] = { 0,2,1,3 };
int state = 0;
public:
Stepperbi(int pin1) {
gpio = pin1;
for (int i = 0; i < 8; i++) {
pinMode(gpio + i, OUTPUT);
digitalWrite(gpio + i, 0);
}
digitalWrite(gpio, 1);
digitalWrite(gpio+2, 1);
}
void step(int step) {
if (step > 0) {
digitalWrite(gpio + stepTable[(state+2) % 4],0);
digitalWrite(gpio + stepTable[state],1);
state = (state + 1) % 4;
} else {
state = (state + 3) % 4;
digitalWrite(gpio + stepTable[(state+2) % 4],1);
digitalWrite(gpio + stepTable[state],0);
};
}
};
Stepperbi stepper1=Stepperbi(16);
void setup(){};
void loop() {
stepper1.step(1);
delay(10);
}
Page 167
Use GPIO 5, 6, 7 and 8 for the Arduino NANO ESP32
Change SPI.begin(14, 12, 13, 15); to SPI.begin(7, 5, 6, 8); and all references to GPIO15 to GPIO8.
Connect pin 12 to 13 for the ESP and 5 to 6 for the NANO ESP32
#include <SPI.h>
void setup() {
pinMode(15, OUTPUT);
digitalWrite(15, 1);
SPI.begin(14, 12, 13, 15);
SPI.beginTransaction(SPISettings(1000000, MSBFIRST, SPI_MODE0));
Serial.begin(9600);
}
void loop() {
digitalWrite(15, 0);
char receivedVal = SPI.transfer(0xAA);
digitalWrite(15, 1);
Serial.println(receivedVal,HEX);
delay(1000);
}
Page 124
Use GPIO 5, 6, 7 and 8 for the Arduino NANO ESP32
Change SPI.begin(14, 12, 13, 15); to SPI.begin(7, 5, 6, 8); and all references to GPIO15 to GPIO8.
#include <SPI.h>
void setup() {
pinMode(15, OUTPUT);
digitalWrite(15, 1);
SPI.begin(14, 12, 13, 15);
SPI.beginTransaction(SPISettings(1000000, MSBFIRST, SPI_MODE0));
Serial.begin(9600);
}
char data[3];
void loop() {
data[0] = 0x01;
data[1] = 0x80;
data[2] = 0x00;
digitalWrite(15, 0);
SPI.transfer(data,3);
digitalWrite(15, 1);
int raw = (data[1] & 0x03) << 8 |data[2];
Serial.println(raw);
float volts=raw*3.3/1023.0;
Serial.println(volts);
delay(1000);
}
Page175
Use GPIO 5, 6, 7 and 8 for the Arduino NANO ESP32
Change SPI.begin(14, 12, 13, 15); to SPI.begin(7, 5, 6, 8); and all references to GPIO15 to GPIO8.
#include <SPI.h>
float readADC(uint8_t chan) {
char data[3];
data[0] = 0x01;
data[1] = (0x08 | chan) << 4;
data[2] = 0x00;
digitalWrite(15, 0);
SPI.transfer(data, 3);
digitalWrite(15, 1);
int raw = (data[1] & 0x03) << 8 | data[2];
float volts = raw * 3.3 / 1023.0;
return volts;
}
void setup() {
pinMode(15, OUTPUT);
digitalWrite(15, 1);
SPI.begin(14, 12, 13, 15);
SPI.beginTransaction(SPISettings(1000000, MSBFIRST, SPI_MODE0));
Serial.begin(9600);
}
void loop() {
float volts = readADC(0);
Serial.println(volts);
delay(1000);
}
Page 182
void setup() {
Serial.begin(9600);
}
void loop() {
int16_t data = analogRead(5);
int32_t volts = analogReadMilliVolts(5);
Serial.println(data);
Serial.println(volts / 1000.0);
}
Page 183
void setup() {
Serial.begin(9600);
}
void loop() {
int16_t data;
unsigned long s = micros();
for (int i = 0; i < 100000; i++) {
data = analogRead(5);
}
s = micros() - s;
Serial.println(s);
s = micros();
for (int i = 0; i < 100000; i++) {
data = analogReadMilliVolts(5);
}
s = micros() - s;
Serial.println(s);
}
Page 185 Not supported on the NANO ESP32
volatile bool adc_coversion_done = false;
void ARDUINO_ISR_ATTR adcComplete(void) {
adc_coversion_done = true;
}
void setup() {
uint8_t adc_pins[] = { 1, 2, 3, 4 };
analogContinuous(adc_pins, 4, 5, 1000, &adcComplete);
analogContinuousStart();
Serial.begin(9600);
}
void loop() {
adc_continuous_data_t *result = NULL;
if (adc_coversion_done == true) {
adc_coversion_done = false;
if (analogContinuousRead(&result, 0)) {
for (int i = 0; i < 4; i++) {
Serial.printf("\nADC PIN %d data:",
result[i].pin);
Serial.printf("\n Avg raw value = %d",
result[i].avg_read_raw);
Serial.printf("\n Avg millivolts value = %d",
result[i].avg_read_mvolts);
}
delay(1000);
}
}
}
Page 186 Note: Only works on an ESP32 or ESP32 S2
void setup() {
}
void loop() {
for (uint8_t i = 0; i < 256; i++) {
dacWrite(25, i);
}
}
Page 187 Note: Only works on an ESP32 or ESP32 S2
void setup() {
}
void loop() {
for (uint8_t i = 0; i < 256; i+= 32) {
dacWrite(25, i);
}
}
Page 187 Note: Only works on an ESP32 or ESP32 S2
uint8_t wave[256];
void setup() {
for (int i = 0; i < 256; i++) {
wave[i] = (uint8_t)((128.0 +
sinf((float)i * 2.0 * 3.14159 / 255.0) * 128.0));
}
}
void loop() {
for (int i = 0; i < 256; i++) {
dacWrite(25, wave[i]);
}
}
Page 190
void setup() {
Serial.begin(9600);
}
void loop() {
int touch = touchRead(4);
Serial.println(touch);
delay(1000);
}
Page 191
void setup() {
Serial.begin(9600);
}
void loop() {
int touch = touchRead(4);
if (touch > 25000) {
Serial.println("pressed");
}
delay(1000);
}
Page 192
void ISR(void){
digitalWrite(2, !digitalRead(2));
}
void setup() {
pinMode(2, OUTPUT);
touchAttachInterrupt(4,ISR,50000);
Serial.begin(9600);
}
void loop() {
}
Page 205
Use GPIO 5, 6 for the Arduino NANO ESP32
Change Wire.setPins(16, 15); tp Wire.setPins(5, 6);
#include "Wire.h"
void setup() {
Serial.begin(9600);
Wire.setPins(16, 15);
Wire.begin();
}
void loop() {
Wire.beginTransmission(0x40);
Wire.write(0xE7);
Wire.endTransmission(true);
Wire.requestFrom(0x40, 1);
char temp = Wire.read();
Serial.printf("User Register = %X \r\n", temp);
delay(2000);
}
Page 207
Use GPIO 5, 6 for the Arduino NANO ESP32
Change Wire.setPins(16, 15); tp Wire.setPins(5, 6);
#include "Wire.h"
void setup() {
Serial.begin(9600);
Wire.setPins(16, 15);
Wire.begin();
}
void loop() {
Wire.beginTransmission(0x40);
Wire.write(0xE3);
Wire.endTransmission();
int count;
count = Wire.requestFrom(0x40, 3);
char msb = Wire.read();
char lsb = Wire.read();
char check = Wire.read();
Serial.printf("\nmsb %d \nlsb %d \nchecksum %d \n",
msb, lsb, check);
delay(2000);
}
Page 209
Use GPIO 5, 6 for the Arduino NANO ESP32
Change Wire.setPins(16, 15); tp Wire.setPins(5, 6);
#include "Wire.h"
void setup() {
Serial.begin(9600);
Wire.setPins(16, 15);
Wire.begin();
}
void loop() {
Wire.beginTransmission(0x40);
Wire.write(0xF3);
Wire.endTransmission();
int count;
do {
count = Wire.requestFrom(0x40, 3);
delay(2);
} while (count == 0);
char msb = Wire.read();
char lsb = Wire.read();
char check = Wire.read();
Serial.printf("\nmsb %d \nlsb %d \nchecksum %d \n",
msb, lsb, check);
delay(2000);
}
Page 210
Use GPIO 5, 6 for the Arduino NANO ESP32
Change Wire.setPins(16, 15); tp Wire.setPins(5, 6);
#include "Wire.h"
void setup() {
Serial.begin(9600);
Wire.setPins(16, 15);
Wire.begin();
}
void loop() {
Wire.beginTransmission(0x40);
Wire.write(0xF3);
Wire.endTransmission();
delay(50);
int count;
count = Wire.requestFrom(0x40, 3);
char msb = Wire.read();
char lsb = Wire.read();
char check = Wire.read();
Serial.printf("\nmsb %d \nlsb %d \nchecksum %d \n",
msb, lsb, check);
delay(2000);
}
Page 213
Use GPIO 5, 6 for the Arduino NANO ESP32
Change Wire.setPins(16, 15); tp Wire.setPins(5, 6);
#include "Wire.h"
uint8_t crcCheck(uint8_t msb, uint8_t lsb, uint8_t check) {
uint32_t data32 = ((uint32_t)msb << 16) | ((uint32_t)lsb << 8) |
(uint32_t)check;
uint32_t divisor = 0x988000;
for (int i = 0; i < 16; i++) {
if (data32 & (uint32_t)1 << (23 - i)) data32 ^= divisor;
divisor >>= 1;
};
return (uint8_t)data32;
}
void setup() {
Serial.begin(9600);
Wire.setPins(16, 15);
Wire.begin();
}
void loop() {
Wire.beginTransmission(0x40);
Wire.write(0xF3);
Wire.endTransmission();
int count;
do {
count = Wire.requestFrom(0x40, 3);
delay(2);
} while (count == 0);
char msb = Wire.read();
char lsb = Wire.read();
char check = Wire.read();
Serial.printf("\nmsb %d \nlsb %d \nchecksum %d \n",
msb, lsb, check);
unsigned int data16 = ((unsigned int)msb << 8) |
(unsigned int)(lsb & 0xFC);
float temp = (float)(-46.85 + (175.72 * data16 / (float)65536));
Serial.printf("Temperature %f C \n", temp);
Wire.beginTransmission(0x40);
Wire.write(0xF5);
Wire.endTransmission(false);
do {
count = Wire.requestFrom(0x40, 3);
delay(2);
} while (count == 0);
msb = Wire.read();
lsb = Wire.read();
check = Wire.read();
Serial.printf("msb %d \n\r lsb %d \n\r checksum %d \n\r",
msb, lsb, check);
data16 = ((unsigned int)msb << 8) | (unsigned int)(lsb & 0xFC);
float hum = -6 + (125.0 * (float)data16) / 65536;
Serial.printf("Humidity %f %% \n\r", hum);
Serial.printf("crc = %d\n\r", crcCheck(msb, lsb, check));
delay(2000);
}
Page 224
void setup() {
Serial.begin(9600);
}
void loop() {
pinMode(2, OUTPUT);
digitalWrite(2, 1);
delay(1000);
digitalWrite(2, 0);
delay(1);
pinMode(2, INPUT);
while (digitalRead(2) == 1) {};
while (digitalRead(2) == 0) {};
while (digitalRead(2) == 1) {};
int64_t t2;
uint32_t data = 0;
int64_t t1 = micros();
for (int i = 0; i < 32; i++) {
while (digitalRead(2) == 0) {
};
while (digitalRead(2) == 1) {
};
t2 = micros();
data = data << 1;
data = data | ((t2 - t1) > 100);
t1 = t2;
}
uint8_t checksum = 0;
for (int i = 0; i < 8; i++) {
while (digitalRead(2) == 0) {};
while (digitalRead(2) == 1) {};
t2 = micros();
checksum = checksum << 1;
checksum = checksum | ((t2 - t1) > 100);
t1 = t2;
}
Serial.printf("data %ld\n", data);
uint8_t byte1 = (data >> 24 & 0xFF);
uint8_t byte2 = (data >> 16 & 0xFF);
uint8_t byte3 = (data >> 8 & 0xFF);
uint8_t byte4 = (data & 0xFF);
Serial.printf("Checksum %X %X\n", checksum,
(byte1 + byte2 + byte3 + byte4) & 0xFF);
float humidity = (float)((byte1 << 8) | byte2) / 10.0;
Serial.printf("Humidity= %f %%\n", humidity);
float temperature;
int neg = byte3 & 0x80;
byte3 = byte3 & 0x7F;
temperature = (float)(byte3 << 8 | byte4) / 10.0;
if (neg > 0)
temperature = -temperature;
Serial.printf("Temperature= %f C\n", temperature);
delay(1000);
}
Page 225
void setup() {
Serial.begin(9600);
}
void loop() {
pinMode(2, OUTPUT);
digitalWrite(2, 1);
delay(1000);
digitalWrite(2, 0);
delay(1);
pinMode(2, INPUT);
while (digitalRead(2) == 1) {};
while (digitalRead(2) == 0) {};
while (digitalRead(2) == 1) {};
uint32_t data = 0;
for (int i = 0; i < 32; i++)
{
while (digitalRead(2) == 0) {};
delayMicroseconds(50);
data = data << 1;
data = data | digitalRead(2);
while (digitalRead(2) == 1) {};
}
uint8_t checksum = 0;
for (int i = 0; i < 8; i++)
{
while (digitalRead(2) == 0) {};
delayMicroseconds(50);
checksum = checksum << 1;
checksum = checksum | digitalRead(2);
while (digitalRead(2) == 1) {};
}
Serial.printf("data %ld\n", data);
uint8_t byte1 = (data >> 24 & 0xFF);
uint8_t byte2 = (data >> 16 & 0xFF);
uint8_t byte3 = (data >> 8 & 0xFF);
uint8_t byte4 = (data & 0xFF);
Serial.printf("Checksum %X %X\n", checksum,
(byte1 + byte2 + byte3 + byte4) & 0xFF);
float humidity = (float)((byte1 << 8) | byte2) / 10.0;
Serial.printf("Humidity= %f %%\n", humidity);
float temperature;
int neg = byte3 & 0x80;
byte3 = byte3 & 0x7F;
temperature = (float)(byte3 << 8 | byte4) / 10.0;
if (neg > 0)
temperature = -temperature;
Serial.printf("Temperature= %f C\n", temperature);
delay(1000);
}
Page 240
uint8_t readBit(uint8_t pin) {
digitalWrite(pin, 0);
delayMicroseconds(2);
digitalWrite(pin, 1);
delayMicroseconds(5);
uint8_t b = digitalRead(pin);
delayMicroseconds(60);
return b;
}
void writeBit(uint8_t pin, int b) {
int delay1, delay2;
if (b == 1) {
delay1 = 6;
delay2 = 64;
} else {
delay1 = 60;
delay2 = 10;
}
digitalWrite(pin, 0);
delayMicroseconds(delay1);
digitalWrite(pin, 1);
delayMicroseconds(delay2);
}
int readByte(uint8_t pin) {
int byte = 0;
for (int i = 0; i < 8; i++) {
byte = byte | readBit(pin) << i;
};
return byte;
}
void writeByte(uint8_t pin, int byte) {
for (int i = 0; i < 8; i++) {
if (byte & 1) {
writeBit(pin, 1);
} else {
writeBit(pin, 0);
}
byte = byte >> 1;
}
}
int presence(int pin) {
pinMode(pin, OUTPUT |OPEN_DRAIN);
digitalWrite(pin, 1);
delayMicroseconds(1000);
digitalWrite(pin, 0);
delayMicroseconds(480);
digitalWrite(pin, 1);
delayMicroseconds(70);
int res = digitalRead(pin);
delayMicroseconds(410);
return res;
}
int convert(uint8_t pin) {
writeByte(pin, 0x44);
int i;
for (i = 0; i < 500; i++) {
delayMicroseconds(10000);
if (digitalRead(pin) == 1)
break;
}
return i;
}
uint8_t crc8(uint8_t* data, uint8_t len) {
uint8_t temp;
uint8_t databyte;
uint8_t crc = 0;
for (int i = 0; i < len; i++) {
databyte = data[i];
for (int j = 0; j < 8; j++) {
temp = (crc ^ databyte) & 0x01;
crc >>= 1;
if (temp)
crc ^= 0x8C;
databyte >>= 1;
}
}
return crc;
}
float getTemperature(uint8_t pin) {
if (presence(pin) == 1) return -1000;
writeByte(pin, 0xCC);
if (convert(pin) == 500) return -3000;
presence(pin);
writeByte(pin, 0xCC);
writeByte(pin, 0xBE);
uint8_t data[9];
for (int i = 0; i < 9; i++) {
data[i] = readByte(pin);
}
uint8_t crc = crc8(data, 9);
if (crc != 0) return -2000;
int t1 = data[0];
int t2 = data[1];
int16_t temp1 = (t2 << 8 | t1);
float temp = (float)temp1 / 16;
return temp;
}
void setup() {
Serial.begin(9600);
}
void loop() {
float temp = getTemperature(2);
Serial.printf("temperature=%f\n", temp);
delay(1000);
}
Page 318 ESP32 S3
void convertGRB(int GRB, rmt_data_t *rawdata) {
int mask = 0x800000;
for (int j = 0; j < 24; j++) {
if ((GRB & mask)) {
rawdata[j].level0 = 1;
rawdata[j].duration0 = 7;
rawdata[j].level1 = 0;
rawdata[j].duration1 = 12 - 7;
} else {
rawdata[j].level0 = 1;
rawdata[j].duration0 = 3;
rawdata[j].level1 = 0;
rawdata[j].duration1 = 12 - 3;
}
mask = mask >> 1;
}
}
rmt_data_t raw_symbols[100];
int pin = 48;
void setup() {
Serial.begin(9600);
rmtInit(pin, RMT_TX_MODE, RMT_MEM_NUM_BLOCKS_1, 10000000);
}
void loop() {
int color = 0x00FFFF;
convertGRB(color, raw_symbols);
rmtWrite(pin, raw_symbols,
24 * sizeof(rmt_data_t), RMT_WAIT_FOR_EVER);
delay(1000);
}
Note GPIO lines used have been changed from 1 and 2 to 12 and 13 to avoid strapping pin.
Page 254
void setup() {
Serial.begin(9600);
Serial1.begin(9600, SERIAL_8N1, 12, 13);
Serial1.setTimeout(30);
}
void loop() {
char buffer[100];
Serial1.write("Hello World\n");
int len = Serial1.readBytes(buffer, 100);
buffer[len] = NULL;
Serial.printf("\nreceived data: %s\n", buffer);
delay(1000);
}
Page 255
void setup() {
Serial.begin(9600);
Serial1.begin(9600, SERIAL_8N1, 12, 13);
Serial1.setTimeout(30);
}
void loop() {
char buffer[100];
Serial1.write("Hello World\n");
if (Serial1.available()) {
char byte;
byte = Serial1.read();
Serial.print(byte);
}
delay(10);
}
Page 257
int count;
char buffer[100]={0};
void recieveData(void){
int nr=Serial1.readBytes(buffer,100);
buffer[nr]=0;
};
void setup() {
Serial.begin(9600);
Serial1.begin(9600, SERIAL_8N1, 12, 13);
Serial1.setTimeout(5);
Serial1.onReceive(recieveData);
}
void loop() {
Serial.print(count++);
Serial.println(buffer);
buffer[0]=0;
Serial1.write("Hello World\n");
delay(2000);
}
Page 258
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial1.setTxBufferSize(256);
Serial1.setRxBufferSize(1024);
Serial1.begin(9600, SERIAL_8N1, 12, 13);
Serial1.setTimeout(30);
int n = 1000;
char SendData[n];
for (int i = 0; i < n; i++) {
SendData[i] = 'A';
}
SendData[n - 1] = 0;
Serial1.flush();
unsigned long s = micros();
Serial1.write(SendData, n);
s = micros() - s;
Serial.printf("\nt= %f\n", s / 1000.0);
Serial.flush();
}
void loop() {
delay(1000);
}
Page 259 - created by adding end on page 259 to previous program
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial1.setTxBufferSize(256);
Serial1.setRxBufferSize(1024);
Serial1.begin(9600, SERIAL_8N1, 12, 13);
Serial1.setTimeout(30);
int n = 1000;
char SendData[n];
for (int i = 0; i < n; i++) {
SendData[i] = 'A';
}
SendData[n - 1] = 0;
Serial1.flush();
unsigned long s = micros();
Serial1.write(SendData, n);
s = micros() - s;
Serial.printf("\nt= %f\n", s / 1000.0);
Serial.flush();
char RecData[n+1];
int nr = Serial1.readBytes(RecData,n+1);
RecData[nr + 1] = 0;
Serial.printf("%s\n", RecData);
Serial.printf("%d %d\n", nr, sizeof(SendData));
}
void loop() {
delay(1000);
}
Page 258
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial1.setTxBufferSize(2048);
Serial1.setRxBufferSize(512);
Serial1.begin(9600, SERIAL_8N1, 12, 13);
Serial1.setTimeout(30);
int n = 1000;
char SendData[n];
for (int i = 0; i < n; i++) {
SendData[i] = 'A' + i / 40;;
}
SendData[n - 1] = 0;
Serial1.flush();
unsigned long s = micros();
Serial1.write(SendData, n);
s = micros() - s;
Serial.printf("t= %f\n", s / 1000.0);
delay(3000);
char RecData[n+1];
int nr = Serial1.readBytes(RecData,n+1);
RecData[nr + 1] = 0;
Serial.printf("%s\n", RecData);
Serial.printf("%d %d\n", nr, sizeof(SendData));
}
void loop() {
delay(1000);
}
Page 264
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial1.setTxBufferSize(2000);
Serial1.setRxBufferSize(130);
Serial1.begin(115200, SERIAL_8N1, 12, 13);
Serial1.setPins(12, 13, 5, 4);
Serial1.setHwFlowCtrlMode(UART_HW_FLOWCTRL_CTS_RTS, 10);
int n = 1000;
char SendData[n];
for (int i = 0; i < n; i++) {
SendData[i] = 'A' + i / 40;
}
SendData[n - 1] = 0;
Serial1.write(SendData, n);
char RecData[2000];
int nr = 0;
int nt = 0;
Serial1.setTimeout(1);
do {
delay(100);
nt = Serial1.readBytes(&RecData[nr], sizeof(RecData) - nr);
nr = nr + nt;
Serial.printf("%d %d\n", nt, nr);
} while (nt != 0);
RecData[nr + 1] = 0;
Serial.printf("%s\n", &RecData[1]);
Serial.printf("%d %d\n", nr, sizeof(SendData));
}
void loop() {
delay(1000);
}
Page 269
int presence() {
Serial1.updateBaudRate(9600);
Serial1.write(0xF0);
char byte;
Serial1.readBytes(&byte, 1);
Serial1.updateBaudRate(115200);
if (byte == 0xF0) return -1;
return 0;
}
void writeByte(int byte) {
char buf[8];
for (int i = 0; i < 8; i++) {
if ((byte & 1) == 1) {
buf[i] = 0xFF;
} else {
buf[i] = 0x00;
}
byte = byte >> 1;
};
Serial1.write(buf, 8);
Serial1.readBytes(buf, 8);
}
char readByte(void) {
char buf[8];
for (int i = 0; i < 8; i++) {
buf[i] = 0xFF;
}
Serial1.write(buf, 8);
Serial1.readBytes(buf, 8);
char result = 0;
for (int i = 0; i < 8; i++) {
result = result >> 1;
if (buf[i] == 0xFF) result = result | 0x80;
}
return result;
}
void setup() {
Serial.begin(9600);
Serial1.begin(115200, SERIAL_8N1, 12, 13);
}
void loop() {
Serial.printf("\n%d\n", presence());
writeByte(0xCC);
writeByte(0x44);
Serial.printf("%d\n", presence());
writeByte(0xCC);
writeByte(0xBE);
char data[9];
for (int i = 0; i < 9; i++) {
data[i] = readByte();
}
int t1 = data[0];
int t2 = data[1];
int temp1 = (t2 << 8 | t1);
if (t2 & 0x80) temp1 = temp1 | 0xFFFF0000;
float temp = temp1 / 16.0;
Serial.printf("temp =%f\n", temp);
delay(2000);
}
Page 274
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include <WiFi.h>
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
int status = WiFi.begin("dlink3", "hawkhawk");
delay(100);
while (WiFi.status() != WL_CONNECTED) {
delay(100);
Serial.println(WiFi.status());
};
}
void loop() {
delay(1000);
}
Page 275
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
The BSSID function doesn't work on the Nano ESP32 and the lines should be removed from the program.
#include <WiFi.h>
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
int status = WiFi.begin("ssid", "password");
delay(100);
while (WiFi.status() != WL_CONNECTED) {
delay(100);
Serial.println(WiFi.status());
};
String ssid = WiFi.SSID();
Serial.printf("ssid %s \n", ssid);
unsigned char bssid[6];
WiFi.BSSID(bssid);
Serial.printf("bssid %X %X %X %X %X %X \n",
bssid[0], bssid[1], bssid[2], bssid[3], bssid[4], bssid[5]);
Serial.printf("RSSI %d\n", WiFi.RSSI());
Serial.print("Local IP ");
Serial.println(WiFi.localIP());
Serial.print("Subnet mask ");
Serial.println(WiFi.subnetMask());
Serial.print("Gateway IP ");
Serial.println(WiFi.gatewayIP());
}
void loop() {
delay(1000);
}
Page 281
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include <WiFi.h>
void WiFiEvent(WiFiEvent_t event) {
Serial.printf("[WiFi-event] event: %d\n", event);
switch (event) {
case ARDUINO_EVENT_WIFI_READY:
Serial.println("WiFi interface ready"); break;
case ARDUINO_EVENT_WIFI_SCAN_DONE:
Serial.println("Completed scan for access points"); break;
case ARDUINO_EVENT_WIFI_STA_START:
Serial.println("WiFi client started"); break;
case ARDUINO_EVENT_WIFI_STA_STOP:
Serial.println("WiFi clients stopped"); break;
case ARDUINO_EVENT_WIFI_STA_CONNECTED:
Serial.println("Connected to access point"); break;
case ARDUINO_EVENT_WIFI_STA_DISCONNECTED:
Serial.println("Disconnected from WiFi access point"); break;
case ARDUINO_EVENT_WIFI_STA_GOT_IP:
Serial.print("Obtained IP address: ");
Serial.println(WiFi.localIP());
break;
case ARDUINO_EVENT_WIFI_STA_LOST_IP:
Serial.println("Lost IP address"); break;
default: break;
}
}
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
WiFi.onEvent(WiFiEvent);
int status = WiFi.begin("ssid", "password");
};
void loop() {
}
Page 282
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include <WiFi.h>
int wifiConnect(char* ssid, char* password) {
int status = WiFi.begin(ssid, password);
while (status != WL_CONNECTED) {
switch (status) {
case WL_NO_SSID_AVAIL:
Serial.printf("No AP with name %s can be found", ssid);
return status;
case WL_CONNECT_FAILED:
Serial.printf("Connection failed");
return status;
case WL_CONNECTION_LOST:
Serial.printf("Connection lost possible security problem");
return status;
}
delay(100);
status = WiFi.status();
}
return status;
}
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
int status=wifiConnect("ssid", "password");
Serial.println(status);
};
void loop(){
delay(1000);
}
Page 282
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include <WiFi.h>
int status;
void WiFiEvent(WiFiEvent_t event) {
Serial.printf("[WiFi-event] event: %d\n", event);
status = event;
switch (event) {
case ARDUINO_EVENT_WIFI_READY:
Serial.println("WiFi interface ready");
break;
case ARDUINO_EVENT_WIFI_SCAN_DONE:
Serial.println("Completed scan for access points");
break;
case ARDUINO_EVENT_WIFI_STA_START:
Serial.println("WiFi client started");
break;
case ARDUINO_EVENT_WIFI_STA_STOP:
Serial.println("WiFi clients stopped");
break;
case ARDUINO_EVENT_WIFI_STA_CONNECTED:
Serial.println("Connected to access point");
break;
case ARDUINO_EVENT_WIFI_STA_DISCONNECTED:
Serial.println("Disconnected from WiFi access point"); break;
case ARDUINO_EVENT_WIFI_STA_GOT_IP:
Serial.print("Obtained IP address: ");
Serial.println(WiFi.localIP());
break;
case ARDUINO_EVENT_WIFI_STA_LOST_IP:
Serial.println("Lost IP address");
break;
default: break;
}
}
void wifiConnect(char* ssid, char* password) {
WiFi.onEvent(WiFiEvent);
status = WiFi.begin(ssid, password);
}
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
WiFi.onEvent(WiFiEvent);
wifiConnect("dlink3", "hawkhawk");
};
void loop() {
while (status != ARDUINO_EVENT_WIFI_STA_GOT_IP) {
delay(1000);
};
Serial.println(status);
delay(5000);
}
Page 284
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include <WiFi.h>
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
int nNet = WiFi.scanNetworks();
Serial.println(nNet);
for (int i = 0; i < nNet; i++) {
Serial.printf("ssid %s rssi %d channel %d encryption %d\n",
WiFi.SSID(i), WiFi.RSSI(i),WiFi.channel(i),
WiFi.encryptionType(i));
}
Serial.println("Scan complete");
};
void loop(){}
Page 385
#include <WiFi.h>
void setup() {
Serial.begin(9600);
WiFi.scanNetworks(true);
};
void loop() {
delay(1000);
int16_t nNet = WiFi.scanComplete();
if (nNet >= 0) {
for (int i = 0; i < nNet; i++) {
Serial.printf("ssid %s rssi %d channel %d encryption %d\n",
WiFi.SSID(i), WiFi.RSSI(i), WiFi.channel(i),
WiFi.encryptionType(i));
}
}
WiFi.scanDelete();
}
Page 287
#include <WiFi.h>
#include <WiFiClient.h>
int wifiConnect(char* ssid, char* password) {
int status = WiFi.begin(ssid, password);
while (status != WL_CONNECTED) {
switch (status) {
case WL_NO_SSID_AVAIL:
Serial.printf("No AP with name %s can be found", ssid);
return status;
case WL_CONNECT_FAILED:
Serial.printf("Connection failed");
return status;
case WL_CONNECTION_LOST:
Serial.printf("Connection lost possible security problem");
return status;
}
delay(100);
status = WiFi.status();
}
return status;
}
WiFiClient client;
void setup() {
Serial.begin(9600);
int status = wifiConnect("dlink3", "hawkhawk");
Serial.println(status);
client.connect("www.example.com", 80);
client.println("GET /index.html HTTP/1.1");
client.println("HOST:example.com");
client.println();
};
void loop() {
if (client.available()) {
char c = client.read();
Serial.print(c);
}
}
Page 289
#include <WiFi.h>
#include <WiFiClientSecure.h>
int wifiConnect(char* ssid, char* password) {
int status = WiFi.begin(ssid, password);
while (status != WL_CONNECTED) {
switch (status) {
case WL_NO_SSID_AVAIL:
Serial.printf("No AP with name %s can be found", ssid);
return status;
case WL_CONNECT_FAILED:
Serial.printf("Connection failed");
return status;
case WL_CONNECTION_LOST:
Serial.printf("Connection lost possible security problem");
return status;
}
delay(100);
status = WiFi.status();
}
return status;
}
WiFiClientSecure client;
void setup() {
Serial.begin(9600);
int status = wifiConnect("ssid", "password");
Serial.println(status);
client.setInsecure();
client.connect("www.example.com", 443);
client.println("GET /index.html HTTP/1.1");
client.println("HOST:example.com");
client.println();
};
void loop() {
if (client.available()) {
char c = client.read();
Serial.print(c);
}
}
Page 292
#include <WiFi.h>
#include <WiFiClient.h>
int wifiConnect(char* ssid, char* password) {
int status = WiFi.begin(ssid, password);
while (status != WL_CONNECTED) {
switch (status) {
case WL_NO_SSID_AVAIL:
Serial.printf("No AP with name %s can be found", ssid);
return status;
case WL_CONNECT_FAILED:
Serial.printf("Connection failed");
return status;
case WL_CONNECTION_LOST:
Serial.printf("Connection lost possible security problem");
return status;
}
delay(100);
status = WiFi.status();
}
return status;
}
WiFiClient client;
void setup() {
Serial.begin(9600);
int status = wifiConnect("ssid", "password");
Serial.println(status);
Serial.print(client.connect("192.168.253.75", 8080));
float temp = 20.5;
int len = snprintf(NULL, 0, "%f", temp);
char *text = (char*)malloc(len + 1);
snprintf(text, len + 1, "%f", temp);
client.println("PUT /index.html HTTP/1.1");
client.println("Content-Type:text/plain");
client.print("Content-Length: ");
client.println(len);
client.println();
client.println(text);
};
void loop() {
if (client.available()) {
char c = client.read();
Serial.print(c);
}
}
Page 293 Python
from http.server import HTTPServer, BaseHTTPRequestHandler
from io import BytesIO
class SimpleHTTPRequestHandler(BaseHTTPRequestHandler):
def log_message(self,*args, **kwargs):
pass
def do_PUT(self):
content_length = int(self.headers['Content-Length'])
body = self.rfile.read(content_length)
bodyString= body.decode(encoding="utf-8")
temp=float(bodyString)
print(temp)
self.send_response(200)
self.end_headers()
httpd = HTTPServer(('', 8080), SimpleHTTPRequestHandler)
httpd.serve_forever()
Page 295
#include <WiFi.h>
#include <WiFiClient.h>
int wifiConnect(char* ssid, char* password) {
int status = WiFi.begin(ssid, password);
while (status != WL_CONNECTED) {
switch (status) {
case WL_NO_SSID_AVAIL:
Serial.printf("No AP with name %s can be found", ssid);
return status;
case WL_CONNECT_FAILED:
Serial.printf("Connection failed");
return status;
case WL_CONNECTION_LOST:
Serial.printf("Connection lost possible security problem");
return status;
}
delay(100);
status = WiFi.status();
}
return status;
}
WiFiServer server(80);
void setup() {
Serial.begin(9600);
int status = wifiConnect("ssid", "password");
Serial.println(status);
server.begin();
};
void loop() {
int buflen = 100;
char buffer[100];
WiFiClient client = server.available();
if (client) {
if (client.connected()) {
Serial.println("connected");
while (client.available()) {
char c = client.read();
Serial.print(c);
}
char page[] = "<html><Body>Temperature =20.5C</Br></body></html>";
int len = strlen(page);
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html; charset=UTF-8");
client.println("Content-Length: ");
client.println(len);
client.println();
client.println(page);
}
}
client.stop();
}
Page 371 Python
with open("iopress.key", 'rb') as f:
lines = f.readlines()
lines=b'"'.join(lines)
lines=lines.decode("ascii")
lines=lines.replace("\n",'\\n"\n')
print("static const unsigned char key[]="+'"', lines+";")
with open("iopress.crt", 'rb') as f:
lines = f.readlines()
lines=b'"'.join(lines)
lines=lines.decode("ascii")
lines=lines.replace("\n",'\\n"\n')
print("static const unsigned char cert[]="+'"', lines+";")
Page 298 HTTPS Server - the certificates listed will eventually timeout and they need to be replaced
#include "esp_https_server.h"
#include <WiFi.h>
int wifiConnect(char* ssid, char* password) {
int status = WiFi.begin(ssid, password);
while (status != WL_CONNECTED) {
switch (status) {
case WL_NO_SSID_AVAIL:
Serial.printf("No AP with name %s can be found", ssid);
return status;
case WL_CONNECT_FAILED:
Serial.printf("Connection failed");
return status;
case WL_CONNECTION_LOST:
Serial.printf("Connection lost possible security problem");
return status;
}
delay(100);
status = WiFi.status();
}
return status;
}
esp_err_t get_handlertemp(httpd_req_t* req)
{
/* Send a simple response */
const char resp[] = "Temperature is 20.3";
httpd_resp_send(req, resp, HTTPD_RESP_USE_STRLEN);
return ESP_OK;
}
esp_err_t get_handlerhum(httpd_req_t* req)
{
/* Send a simple response */
const char resp[] = "Humidity is 80%";
httpd_resp_send(req, resp, HTTPD_RESP_USE_STRLEN);
return ESP_OK;
}
void setup() {
Serial.begin(9600);
int status = wifiConnect("dlink3", "hawkhawk");
Serial.println(status);
static const unsigned char cert[] = " -----BEGIN CERTIFICATE-----\n"
"MIIDazCCAlOgAwIBAgIUA+lvUf9wMrNvaz9DuKnfx4TCoeQwDQYJKoZIhvcNAQEL\n"
"BQAwRTELMAkGA1UEBhMCR0IxEzARBgNVBAgMClNvbWUtU3RhdGUxITAfBgNVBAoM\n"
"GEludGVybmV0IFdpZGdpdHMgUHR5IEx0ZDAeFw0yNDA4MTYxMzQwMTNaFw0yNTA4\n"
"MTYxMzQwMTNaMEUxCzAJBgNVBAYTAkdCMRMwEQYDVQQIDApTb21lLVN0YXRlMSEw\n"
"HwYDVQQKDBhJbnRlcm5ldCBXaWRnaXRzIFB0eSBMdGQwggEiMA0GCSqGSIb3DQEB\n"
"AQUAA4IBDwAwggEKAoIBAQC5zxoZHid/tAtRY+V/Y1rRue4yMiHVLBTmh0kqGM/h\n"
"NvOuxJUnXKP2qn9cbM1OhZvF7NIIcqNTfHaNwDG+tF8p7YlQGcSBdk5+v3HTIAFI\n"
"gg3nwHEWdhNhfNnyHrjJG4YDkLGR9KZwMFfBYpsQJHwegUEpYG+5HnaMncjsJu2Q\n"
"bSO7fQ9dSBC7tIidfv6DhWdz/dHGjqpWYRwHhPACgwS1kKjWiOSrUMWUm3T3px7p\n"
"UfND7Ypz4/1ObTNZJs8zV8bnWp68YxS0rAeD0QIX3yTgvAGV56Dqdl2V4V5D/dpR\n"
"No99W3oUu99YeAK/t5pL+Xu5aXDdeg2e1OhPW7o9fZnlAgMBAAGjUzBRMB0GA1Ud\n"
"DgQWBBQQ4grGsqpNnsjZhWuWOg/Cey7AtTAfBgNVHSMEGDAWgBQQ4grGsqpNnsjZ\n"
"hWuWOg/Cey7AtTAPBgNVHRMBAf8EBTADAQH/MA0GCSqGSIb3DQEBCwUAA4IBAQAj\n"
"lw6xqyLBB86XIpW1YAINVHEw9x5ewMSbYTN7pDj01tRlaLfr8S4Qvo4FBA2Mq5fn\n"
"fstJzucb18yX15ZNo6xD1fAPYRf6BK6UwSeo/U4Hjewkk7gOyKEW8IjAMtWB5Svr\n"
"bZn4wxcSZEX/EHtGWe0kCZ4bDlWn9GuSjtAIZcrKo+jr0Cos2O4t19MWgxrbOwMx\n"
"AJYepL5+YcMd0NPuERBfTHE7mIG1heH8DAXAHtnFw26835aEyseZIR6EYPVxESYI\n"
"xmszSRgHFWDEqPpvaFpdOT1fT4KsloSP+wkE8DcmvjYRbPeabc4z8vTHRWgyLsHJ\n"
"mjqAoUl1y8um2Iw5ko0N\n"
"-----END CERTIFICATE-----\n";
static const unsigned char key[] = " -----BEGIN PRIVATE KEY-----\n"
"MIIEvQIBADANBgkqhkiG9w0BAQEFAASCBKcwggSjAgEAAoIBAQC5zxoZHid/tAtR\n"
"Y+V/Y1rRue4yMiHVLBTmh0kqGM/hNvOuxJUnXKP2qn9cbM1OhZvF7NIIcqNTfHaN\n"
"wDG+tF8p7YlQGcSBdk5+v3HTIAFIgg3nwHEWdhNhfNnyHrjJG4YDkLGR9KZwMFfB\n"
"YpsQJHwegUEpYG+5HnaMncjsJu2QbSO7fQ9dSBC7tIidfv6DhWdz/dHGjqpWYRwH\n"
"hPACgwS1kKjWiOSrUMWUm3T3px7pUfND7Ypz4/1ObTNZJs8zV8bnWp68YxS0rAeD\n"
"0QIX3yTgvAGV56Dqdl2V4V5D/dpRNo99W3oUu99YeAK/t5pL+Xu5aXDdeg2e1OhP\n"
"W7o9fZnlAgMBAAECggEABezu3iIyDEaHnd7bsMZQXSPazsr+fTfcqsVhte/4oSwJ\n"
"dWdbglfX+sPRL/dgTMLCBvvYbuCJCN6NQVQBwh0qc8HZgS5xL9fABRbB4IPCxrcv\n"
"Dlb6xEabs54xrSEBr5grG+3/W7I7pJRGGCq22zruomJo25LxvSuViEJ35+AN728d\n"
"rQW4qce1w0837O15u5EnWVrtEtFbvZHytuywvrGKDkB5mmoCE+31ASPnVH3iQFjw\n"
"s0HPmMufHfKRKnlucfCl5jJToHxIHf4JBd1LlvjpJfi0EtjLNbxg9vhCr4roKdqq\n"
"BvM+btu31X7Q/R0Ne3p7V5J3FRNwA3Bce7P6TVsqAQKBgQDdi+5e3FR8QY/qJ9xk\n"
"4MnxycBhcpd2Wtk7oqCs314fk0ifxtQHUh3QKPmTnqJiwxGRdYje05JHHCo2bgMb\n"
"FN7AHw1NsYSfsxqYxpgkovc1HJxKNbcqfI/bC1q1lT3Vt9gGk7DCD73EauVIAwOe\n"
"ybfpqte0Ej95zzTAWqRzw3JmQQKBgQDWtGiC/82qJ7X7xwpjnP0++RauF+TC71Hy\n"
"alqgTbwy0SEaGcxSdGMaxHKK0p94BIs3ZrSsSXLLUSrYC2c0UYnzFLT2i/uJD4NY\n"
"NrD4Xwq1Wo6vWLvY2EU618nTFmzDGOaC1enA030puRGRWEH+35iud7AIyuuPyLhr\n"
"Ek0zNIkypQKBgG9UUwu+QoJSW+R59WmIAFMNZCxT7kLeck1icsWMVXsegx8vRfsL\n"
"y8l/3bLNw6JHjjt/SbFXtikfwSKq88qXGTyIHiJNs2yhDxt4qJm4futknjE4fvvN\n"
"rmiPcxzOi00rXlYnv2o1iNH8OY2PXjFcApxcapqllNo8QrDqm7tEmudBAoGAcNua\n"
"CCoQYH3JQhSJGH1//OcQDekPXYxQ5f0TsCnMYGXfYYnoBfuZ0Issrl4yZvL0fuWk\n"
"2N8u0ULUI4Yy9KRbwAPFb8d7K7uUzfzJn3TN+zAjynX5H+3mzhx5wVSLTS48lM9+\n"
"tNY2d4UJf/4FisTby/Gr/aM0mXrnvZh8LgtShuUCgYEAw+3K2PalVraWHGbg18pz\n"
"fL212YObHDdbHM+SaBoopuiJed9Yz5DRbhVSSfuJMNu9WMjk9aR3Tr7s41l5L/+M\n"
"YGJGNJCE7I4mIfvgXwezxgd5P39+2Ei/qwR9nwsX/y6Mp3EuLKuPJUUaZERjrkIl\n"
"EVzn7XZ781QWSSBer5/vcQM=\n"
"-----END PRIVATE KEY-----\n";
httpd_ssl_config_t config = HTTPD_SSL_CONFIG_DEFAULT();
config.servercert = cert;
config.servercert_len = sizeof(cert);
config.prvtkey_pem = key;
config.prvtkey_len = sizeof(key);
httpd_handle_t server = NULL;
httpd_uri_t uri_get = {
.uri = "/temp",
.method = HTTP_GET,
.handler = get_handlertemp,
.user_ctx = NULL
};
if (httpd_ssl_start(&server, &config) == ESP_OK) {
httpd_register_uri_handler(server, &uri_get);
uri_get.uri = "/hum";
uri_get.handler = get_handlerhum;
httpd_register_uri_handler(server, &uri_get);
}
};
void loop() {
}
Page 304
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial.println();
esp_partition_iterator_t partit =
esp_partition_find(ESP_PARTITION_TYPE_DATA,
ESP_PARTITION_SUBTYPE_ANY, NULL);
while (partit != NULL) {
const esp_partition_t* part = esp_partition_get(partit);
Serial.printf("DATA label= %s Address= %lX size = %ld \n",
part->label, part->address, part->size);
partit = esp_partition_next(partit);
};
esp_partition_iterator_release(partit);
Serial.printf("\n");
partit = esp_partition_find(ESP_PARTITION_TYPE_APP,
ESP_PARTITION_SUBTYPE_ANY, NULL);
while (partit != NULL) {
const esp_partition_t* part = esp_partition_get(partit);
Serial.printf("APP label= %s Address= %lX size = %ld \n",
part->label, part->address, part->size);
partit = esp_partition_next(partit);
};
esp_partition_iterator_release(partit);
}
void loop() {
}
Page 307
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include "nvs_flash.h"
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial.println();
nvs_flash_init();
nvs_handle_t my_handle;
nvs_open("localstorage", NVS_READWRITE, &my_handle);
char key[] = "mykey";
int32_t myvalue = 42;
int32_t myretrievedvalue;
nvs_set_i32(my_handle, key, myvalue);
nvs_commit(my_handle);
nvs_get_i32(my_handle, key, &myretrievedvalue);
Serial.printf("stored value = %ld\n", myretrievedvalue);
}
void loop(){}
Page 308
#include "FS.h"
#include "FFat.h"
void setup() {
Serial.begin(9600);
Serial.println();
Serial.println(FFat.format(true,"ffat"));
}
void loop() {
}
Page 309 Complete program
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include "FS.h"
#include "FFat.h"
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial.println();
FFat.begin(false, "", 2, "ffat");
Serial.printf("Total space: %10u\n", FFat.totalBytes());
Serial.printf("Free space: %10u\n", FFat.freeBytes());
File f = FFat.open("/hello.txt", FILE_WRITE);
f.println("Hello world");
f.close();
f = FFat.open("/hello.txt",FILE_READ);
while (f.available()) {
char c = f.read();
Serial.write(c);
}
f.close();
Serial.println("File Closed");
}
void loop() {}
Page 310
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include "FS.h"
#include "FFat.h"
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial.println();
FFat.begin(false, "", 2, "ffat");
Serial.printf("Total space: %10u\n", FFat.totalBytes());
Serial.printf("Free space: %10u\n", FFat.freeBytes());
File root=FFat.open("/");
File file = root.openNextFile();
while(file){
Serial.println(file.name());
file = root.openNextFile();
}
}
void loop() {
}
Page 313
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
#include "FS.h"
#include "SD.h"
#include "SPI.h"
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial.println();
SPI.begin(18, 4, 15, 5);
SD.begin(5);
uint64_t cardSize = SD.cardSize() / (1024 * 1024);
Serial.printf("SD Card Size: %lluMB\n", cardSize);
File f = SD.open("/hello.txt", FILE_WRITE);
f.println("Hello world");
f.close();
f = SD.open("/hello.txt",FILE_READ);
while (f.available()) {
char c = f.read();
Serial.write(c);
}
f.close();
Serial.println("File Closed");
}
void loop() {
}
Page 317
uint32_t* GPIObase = (uint32_t*)0x60004000; //EP32 S3
//uint32_t* GPIObase = (uint32_t*)0x3FF44000; //ESP32
uint32_t* GPIOSet = GPIObase + 8 / 4;
uint32_t* GPIOClear = GPIObase + 0xC / 4;
uint32_t mask = 1 << 2;
void setup() {
pinMode(2, OUTPUT);
}
void loop() {
*GPIOSet = mask;
delay(1000);
*GPIOClear = mask;
delay(1000);
}
Page 318
#include "soc/gpio_reg.h"
uint32_t mask = 1 << 2;
void setup() {
pinMode(2, OUTPUT);
}
void loop() {
*(int32_t*)GPIO_OUT_W1TS_REG = mask;
delay(1000);
*(int32_t*)GPIO_OUT_W1TC_REG = mask;
delay(1000);
}
Path 320
#include "soc/gpio_reg.h"
uint32_t mask = (1 << 2) | (1 << 4);
uint32_t value1 = (1 << 2);
uint32_t value2 = (1 << 4);
void gpio_value_mask(int32_t value, int32_t mask) {
*(int32_t*)GPIO_OUT_REG = (*(int32_t*)GPIO_OUT_REG & ~mask) | (value & mask);
}
void setup() {
pinMode(2, OUTPUT);
pinMode(4, OUTPUT);
}
void loop() {
gpio_value_mask(value1, mask);
gpio_value_mask(value2, mask);
}
Path 322
Does not work on NANO ESP32
bool isrollover(int timer) {
int32_t *base=(int32_t*)0x600190C0; //ESP32 S3
//int32_t *base=(int32_t*)0x3FF59180; //ESP32
bool value = *((int32_t*)base) & 1<<timer;
*((int32_t*)base+3) = 1<<timer;
return value;
}
uint8_t wave[256];
void setup() {
ledcAttach(2, 150000, 8);
for (int i = 0; i < 256; i++) {
wave[i] = (uint8_t)((128.0 +
sinf((float)i * 2.0 * 3.14159 / 255.0) * 128.0));
}
}
void loop() {
for (int i = 0; i < 256; i++) {
analogWrite(2, wave[i]);
while (!isrollover(0)) {};
}
}
Page 325
Note change the start of setup to:
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
to setup to force some ESP32s to change baud rate after upload and before calling loop.
Does not work on NANO ESP32 as the header file is not installed.
#include "esp_netif_sntp.h"
#include <WiFi.h>
int wifiConnect(char* ssid, char* password) {
int status = WiFi.begin(ssid, password);
while (status != WL_CONNECTED) {
switch (status) {
case WL_NO_SSID_AVAIL:
Serial.printf("No AP with name %s can be found", ssid);
return status;
case WL_CONNECT_FAILED:
Serial.printf("Connection failed");
return status;
case WL_CONNECTION_LOST:
Serial.printf("Connection lost possible security problem");
return status;
}
delay(100);
status = WiFi.status();
}
return status;
}
void setup() {
Serial.begin(9600);
delay(2000);
Serial.begin(9600);
Serial.println();
int status = wifiConnect("dlink3", "hawkhawk");
Serial.println(status);
esp_sntp_config_t config =
ESP_NETIF_SNTP_DEFAULT_CONFIG("pool.ntp.org");
esp_netif_sntp_init(&config);
if (esp_netif_sntp_sync_wait(pdMS_TO_TICKS(10000)) != ESP_OK)
{
printf("Failed to update system time within 10s timeout");
}
struct timeval t;
gettimeofday(&t, NULL);
setenv("TZ", "UTC-1", 1);
tzset();
struct tm *tm = localtime(&(t.tv_sec));
printf("hour = %d\n", tm->tm_hour);
printf("min = %d\n", tm->tm_min);
char date[100];
strftime(date, 100, "%a, %d %b %Y %T", tm);
printf("date = %s\n", date);
}
void loop() {
}
Page 328
Does not work on NANO ESP32 because of problem restarting USB serial connection
void setup() {
Serial.begin(9600);
Serial.println();
}
void loop() {
esp_sleep_enable_timer_wakeup(1000000);
for (int i = 0; i < 10; i++) {
Serial.printf("starting sleep %d \n", i);
Serial.flush();
esp_light_sleep_start();
Serial.printf("back from sleep %d \n", i);
}
}
Page 330
Does not work on NANO ESP32 because of problem restarting USB serial connection
void setup() {
Serial.begin(9600);
Serial.println();
}
void loop() {
esp_sleep_enable_timer_wakeup(1000000);
for (int i = 0; i < 10; i++) {
Serial.printf("starting sleep %d \n", i);
Serial.flush();
esp_deep_sleep_start();
Serial.printf("back from sleep %d \n", i);
}
}
Page 340
void task1(void* arg) {
for (;;) {
digitalWrite(2, 1);
}
}
void task2(void* arg) {
for (;;) {
digitalWrite(2, 0);
}
}
void setup() {
Serial.begin(9600);
Serial.println();
pinMode(2, OUTPUT);
vTaskSuspendAll();
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048, NULL, 1, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 2048, NULL, 1, &th2, 1);
xTaskResumeAll();
}
void loop() {
}
Page 344
uint64_t flag1 = 0;
uint64_t flag2 = 0;
void task1(void* arg) {
for (;;) {
flag1 = 0xFFFFFFFFFFFFFFFF;
flag2 = 0xFFFFFFFFFFFFFFFF;
if (flag1 != flag2) {
Serial.printf("task 1 %llX %llX\n", flag1, flag2);
}
}
}
void task2(void* arg) {
for (;;) {
flag1 = 0x0;
flag2 = 0x0;
if (flag1 != flag2) {
Serial.printf("task 2 %llX %llX\n", flag1, flag1);
}
}
}
void setup() {
Serial.begin(9600);
Serial.println();
vTaskSuspendAll();
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048, NULL, 1, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 2048, NULL, 1, &th2, 1);
xTaskResumeAll();
}
void loop() {
}
Page 347
int64_t count = 0;
void task1(void* arg) {
for (int i = 0;i < 0xFFFFF; i++) {
count = count + 1;
}
for (;;) {
}
}
void task2(void* arg) {
for (int i = 0;i < 0xFFFFF; i++) {
count = count + 1;
}
for (;;) {
}
}
void setup() {
Serial.begin(9600);
Serial.println();
vTaskSuspendAll();
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048, NULL, 1, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 2048, NULL, 0, &th2, 0);
xTaskResumeAll();
delay(4000);
Serial.printf("%llX\n", count);
}
void loop() {
}
Page 349
int64_t count = 0;
static portMUX_TYPE my_spinlock = portMUX_INITIALIZER_UNLOCKED;
void task1(void* arg) {
for (int i = 0;i < 0xFFFFF; i++) {
taskENTER_CRITICAL(&my_spinlock);
count = count + 1;
taskEXIT_CRITICAL(&my_spinlock);
}
for (;;) {
}
}
void task2(void* arg) {
for (int i = 0;i < 0xFFFFF; i++) {
taskENTER_CRITICAL(&my_spinlock);
count = count + 1;
taskEXIT_CRITICAL(&my_spinlock);
}
for (;;) {
}
}
void setup() {
Serial.begin(9600);
Serial.println();
vTaskSuspendAll();
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048, NULL, 1, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 2048, NULL, 0, &th2, 0);
xTaskResumeAll();
delay(4000);
Serial.printf("%llX\n", count);
}
void loop() {
}
Page
QueueHandle_t q;
int64_t count = 0;
void task1(void* arg) {
for (;;) {
xQueueSendToBack(q, &count, 2);
count++;
vTaskDelay(1);
}
}
void task2(void* arg) {
int64_t data;
for (;;) {
xQueueReceive(q, &data, 20);
Serial.printf("%llX %d\n", data,uxQueueSpacesAvailable(q));
}
}
void setup() {
Serial.begin(9600);
Serial.println();
q = xQueueCreate(100, sizeof(int64_t));
vTaskSuspendAll();
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048, NULL, 1, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 4048, NULL, 1, &th2, 1);
xTaskResumeAll();
}
void loop() {
}
Page 354
Not supported on NANO ESP32
#include "esp_task_wdt.h"
esp_task_wdt_user_handle_t uhandle;
void setup() {
Serial.begin(9600);
Serial.println();
esp_task_wdt_add_user("TestWD", &uhandle);
}
void loop() {
esp_task_wdt_reset_user(uhandle);
delay(10);
}